Originally published on medium.com
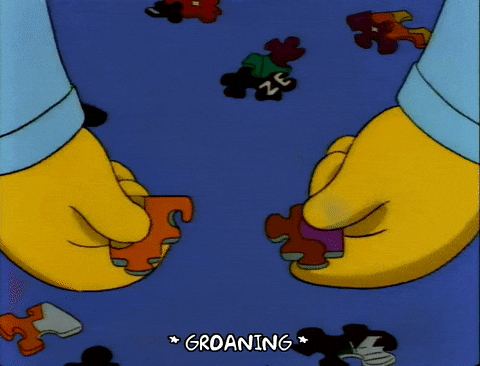
Introduction
Having recently integrated CCAvenue Payment Gateway into my Node + Angular Application, I felt the need to share my experiences as there is a lack of technical help and content in this ever evolving world.
Firstly, this is not a guide on how to integrate the payment gateway, it is more to help you on how to set this up in a Node + Angular (Webpack) environment. It will define the interaction between our JavaScript App and the CCAvenue API
This article should help you integrating the NONSEAMLESS Payment Gateway
While you may find a lot of examples and help for Angular.js (1.x.x), This write up will set you up for Angular 2+
Let’s break up the Payment process into 2 parts
- PART 1. Sending the request
- PART 2. Receiving the response
PART 1. Sending the request
To send the request, we need to redirect to the CCAvenue Test or Production URL with the encrypted details via a POST method.
<form
id="nonseamless"
method="post"
name="redirect"
action="https://test.ccavenue.com/transaction/transaction.do?command=initiateTransaction"
/>
<input type="hidden" id="encRequest" name="encRequest" value="' + encRequest + '">
<input type="hidden" name="access_code" id="access_code" value="' + accessCode + '">
</form>
In a traditional Ejs or HTML scenario, it is easy to go ahead with the <form/>
tag, Encrypt the details server side and then hit the CCAvenue URL from the Node server itself. But, in Angular, the Forms are template driven and are automatically tagged as NgForm & the ANGULAR ROUTING is a different game altogether! Hence there are 2 problems,
- Angular does not let us redirect to the URL with POST method with form input due to NgForm.
- And since the routing is handled by Angular, Node will not route to the above Form action URL given on ccavenuerequest.html gist embedded above.
Solution
Once you are redirecting to the App Payment Route in your Angular App, Get your encRequest variable and bind it with Angular variable. In the Component HTML (payment.component.html), you can see the value binding.
We use ngNoForm as it turns off the ngSubmit behaviour
<div class="container">
<div class="row">
<h1>Proceed to Pay</h1>
<button (click)="pay()"></button>
</div>
</div>
<form #form ngNoForm
id="nonseamless"
method="post"
name="redirect"
action="https://test.ccavenue.com/transaction/transaction.do?command=initiateTransaction">
<input type="hidden" id="encRequest" name="encRequest" [ngModel]="encRequest">
<input type="hidden" name="access_code" id="access_code" [ngModel]="accessCode">
</form>
The TypeScript File should get the encrypted request which is supposed to be sent to the CCAvenue URL in the POST method. The encrypted data will be generated by Node using the crypto Node module based on the url encoded request parameters
import { Component, OnInit, ElementRef, ViewChild } from '@angular/core';
@Component({
selector: '...',
templateUrl: '...',
styleUrls: [...],
providers: [...]
})
export class PaymentComponent implements OnInit {
@ViewChild('form') form: ElementRef;
encRequest: String;
accessCode: String;
ngOnInit() {
this.accessCode = 'YOURACCESSCODEGOESHERE';
}
pay() {
// this.cartValue contains all the order information which is sent to the server
// You can use this package to encrypt - https://www.npmjs.com/package/node-ccavenue/
this.checkoutService.getEnc(this.orderInformation).subscribe((response: any) => {
this.encRequest = response.encRequest;
setTimeout(_ => this.form.nativeElement.submit());
}, error => {
console.log(error);
});
}
}
This shall enable you to successfully redirect to the CCAvenue Test or Production Link where the user can proceed with the payment.
PART 2. Receiving the response
You should keep your Redirect or Cancel URL back to the Node URL (This is where your App APIs live), Remember, not the Angular URLs which are routed by the browser. The URL should be capable of handling a POST request. The POST request is called by CCAvenue once the payment is complete with the encrypted details of payment (Success or Failure & Other Params)
const nodeCCAvenue = require('node-ccavenue');
const ccav = new nodeCCAvenue.Configure({
merchant_id: process.env.test_merchant_id || process.env.prod_merchant_id,
working_key: process.env.test_working_key || process.env.prod_working_key
});
router.post('/handleccavenueresponse', (req,res) => {
const { encResp } = req.body;
const output = ccav.redirectResponseToJson(encResp);
logger.log(output);
// The 'output' variable is the CCAvenue Response in JSON Format
if(output.order_status === 'Failure') {
// DO YOUR STUFF
res.writeHead(301,
{Location: 'https://yoururlgoeshere.com/client-side-failureroute'}
);
res.end();
} else if (output.order_status === 'Success') {
// DO YOUR STUFF
res.writeHead(301,
{Location: 'https://yoururlgoeshere.com/client-side-successroute'}
);
res.end();
})
}
})
Aaand you’re done!
Update: 15th April 2019
Based on a lot of requests, I have made a node module to handle the server side encryption/decryption for using CCAvenue with Node.js.
I personally use this module for my projects which use CCAvenue.
If you find you have improvements for the npm package which may benefit others, write me an email or go ahead create a pull request with the changes
https://www.npmjs.com/package/node-ccavenue
Encapsulating the overall process
- Backend — Generate the encoded request parameters (encRequest) based on the user’s purchase preferences (create an order)
- Frontend — Load encRequest and submit the CCAvenue POST + Redirect form (using ngNoForm)
- Payment Gateway — User completes the payment
- Backend — Handle the CCAvenue Response on your Server Side route, decode the response, update your database and redirect to your Client Side route.